Find and display pointing nodes using lstmcpipe#
[1]:
import lstmcpipe
print(f"lstmcpipe v{lstmcpipe.__version__}")
lstmcpipe v0.10.0
[2]:
import os
import matplotlib.pyplot as plt
from lstmcpipe.config import paths_config
Creating a PathConfig#
In lstmcpipe, a PathConfig handles the paths to the different data levels, models, etc… that will then help orchestrate all the jobs and their dependencies.
We need to create one to load the existing poitings. To do so, you need to run the following on the cluster at La Palma.
You will find different PathConfig in the paths_config
module depending on what you want to do. Here we’ll use the PathConfigAllSkyFull
one, that can orchestrate a complete analysis for the AllSky production.
[3]:
paths_config.PathConfigAllSkyFull?
Init signature: paths_config.PathConfigAllSkyFull(prod_id, dec_list)
Docstring: Base class to generate a Path configuration for a production
Init docstring:
Does training and testing for a list of declinations
Parameters
----------
prod_id: str
dec_list: [str]
File: /fefs/aswg/workspace/thomas.vuillaume/software/miniconda3/envs/lstmcpipe/lib/python3.7/site-packages/lstmcpipe/config/paths_config.py
Type: type
Subclasses: PathConfigAllSkyFullDL1ab
NB: The dec_list
is used for the training nodes only. There will be as many models trained as declination provided.
The available declinations can be found here:
[4]:
dec_list = os.listdir("/fefs/aswg/data/mc/DL0/LSTProd2/TrainingDataset/Protons")
dec_list
[4]:
['dec_6166',
'dec_min_2924',
'dec_931',
'dec_3476',
'dec_6676',
'dec_min_413',
'dec_2276',
'dec_4822',
'dec_min_1802']
[5]:
config = paths_config.PathConfigAllSkyFull('all_decs', dec_list)
Training configs#
There is one PathConfigAllSkyTraining
per pointing:
[6]:
config.train_configs
[6]:
{'dec_6166': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da1810>,
'dec_min_2924': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da14d0>,
'dec_931': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da1750>,
'dec_3476': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da1450>,
'dec_6676': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da1690>,
'dec_min_413': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da1390>,
'dec_2276': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da15d0>,
'dec_4822': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da1310>,
'dec_min_1802': <lstmcpipe.config.paths_config.PathConfigAllSkyTraining at 0x7fecc8da1910>}
For each of them, one can access the config, and in particular the pointings
[7]:
config.train_configs['dec_2276'].pointings
[7]:
alt | az | dirname_GammaDiffuse | dirname_Protons |
---|---|---|---|
deg | deg | ||
float64 | float64 | str37 | str29 |
23.554000000000002 | 75.983 | node_corsika_theta_66.446_az_75.983_ | node_theta_66.446_az_75.983_ |
23.554000000000002 | 284.017 | node_corsika_theta_66.446_az_284.017_ | node_theta_66.446_az_284.017_ |
30.658 | 79.18 | node_corsika_theta_59.342_az_79.180_ | node_theta_59.342_az_79.180_ |
30.658 | 280.82 | node_corsika_theta_59.342_az_280.820_ | node_theta_59.342_az_280.820_ |
37.838 | 82.384 | node_corsika_theta_52.162_az_82.384_ | node_theta_52.162_az_82.384_ |
37.838 | 277.616 | node_corsika_theta_52.162_az_277.616_ | node_theta_52.162_az_277.616_ |
45.073 | 85.716 | node_corsika_theta_44.927_az_85.716_ | node_theta_44.927_az_85.716_ |
45.073 | 274.284 | node_corsika_theta_44.927_az_274.284_ | node_theta_44.927_az_274.284_ |
52.339 | 89.359 | node_corsika_theta_37.661_az_89.359_ | node_theta_37.661_az_89.359_ |
52.339 | 270.641 | node_corsika_theta_37.661_az_270.641_ | node_theta_37.661_az_270.641_ |
59.61 | 93.64 | node_corsika_theta_30.390_az_93.640_ | node_theta_30.390_az_93.640_ |
59.61 | 266.36 | node_corsika_theta_30.390_az_266.360_ | node_theta_30.390_az_266.360_ |
66.839 | 99.261 | node_corsika_theta_23.161_az_99.261_ | node_theta_23.161_az_99.261_ |
66.839 | 260.739 | node_corsika_theta_23.161_az_260.739_ | node_theta_23.161_az_260.739_ |
73.913 | 108.09 | node_corsika_theta_16.087_az_108.090_ | node_theta_16.087_az_108.090_ |
73.913 | 251.91 | node_corsika_theta_16.087_az_251.910_ | node_theta_16.087_az_251.910_ |
80.42099999999999 | 126.888 | node_corsika_theta_9.579_az_126.888_ | node_theta_9.579_az_126.888_ |
80.42099999999999 | 233.112 | node_corsika_theta_9.579_az_233.112_ | node_theta_9.579_az_233.112_ |
84.0 | 180.0 | node_corsika_theta_6.000_az_180.000_ | node_theta_6.000_az_180.000_ |
One can display them:
[8]:
config.train_configs['dec_2276'].plot_pointings()
[8]:
<PolarAxesSubplot:xlabel='Azimuth'>
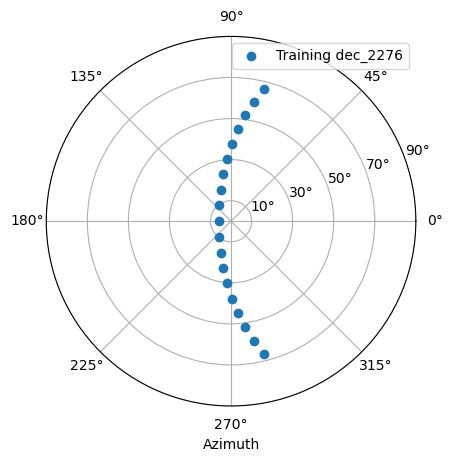
Test config#
One can do the same for the testing nodes.
There is also one config per test, since each trained model will be applied to the test nodes to produce the dedicated IRFs
[9]:
config.test_configs
[9]:
{'dec_6166': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da1510>,
'dec_min_2924': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da12d0>,
'dec_931': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da1950>,
'dec_3476': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da1990>,
'dec_6676': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da19d0>,
'dec_min_413': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da1a10>,
'dec_2276': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da1a50>,
'dec_4822': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da1a90>,
'dec_min_1802': <lstmcpipe.config.paths_config.PathConfigAllSkyTesting at 0x7fecc8da1ad0>}
[10]:
config.test_configs['dec_2276'].pointings
[10]:
alt | az | dirname |
---|---|---|
deg | deg | |
float64 | float64 | str29 |
16.858000000000004 | 331.979 | node_theta_73.142_az_331.979_ |
19.268 | 330.659 | node_theta_70.732_az_330.659_ |
46.803 | 87.604 | node_theta_43.197_az_87.604_ |
21.932000000000002 | 283.075 | node_theta_68.068_az_283.075_ |
37.626 | 216.698 | node_theta_52.374_az_216.698_ |
29.472 | 175.158 | node_theta_60.528_az_175.158_ |
29.340000000000003 | 327.483 | node_theta_60.66_az_327.483_ |
37.626 | 197.973 | node_theta_52.374_az_197.973_ |
37.626 | 110.312 | node_theta_52.374_az_110.312_ |
75.016 | 175.158 | node_theta_14.984_az_175.158_ |
52.186 | 90.0 | node_theta_37.814_az_90_ |
46.803 | 230.005 | node_theta_43.197_az_230.005_ |
32.005 | 327.238 | node_theta_57.995_az_327.238_ |
46.803 | 175.158 | node_theta_43.197_az_175.158_ |
80.0 | 248.117 | node_theta_10.0_az_248.117_ |
54.096 | 17.462 | node_theta_35.904_az_17.462_ |
21.932000000000002 | 231.243 | node_theta_68.068_az_231.243_ |
... | ... | ... |
50.354 | 335.667 | node_theta_39.646_az_335.667_ |
14.774000000000001 | 213.73 | node_theta_75.226_az_213.73_ |
21.932000000000002 | 175.158 | node_theta_68.068_az_175.158_ |
46.803 | 120.311 | node_theta_43.197_az_120.311_ |
26.744999999999997 | 327.961 | node_theta_63.255_az_327.961_ |
7.844999999999999 | 271.199 | node_theta_82.155_az_271.199_ |
66.37 | 259.265 | node_theta_23.630_az_259.265_ |
57.941 | 136.053 | node_theta_32.059_az_136.053_ |
21.715999999999994 | 329.555 | node_theta_68.284_az_329.555_ |
37.626 | 175.158 | node_theta_52.374_az_175.158_ |
14.774000000000001 | 31.342 | node_theta_75.226_az_31.342_ |
37.626 | 240.004 | node_theta_52.374_az_240.004_ |
21.932000000000002 | 141.683 | node_theta_68.068_az_141.683_ |
29.340000000000003 | 32.517 | node_theta_60.66_az_32.517_ |
21.932000000000002 | 119.073 | node_theta_68.068_az_119.073_ |
37.626 | 152.343 | node_theta_52.374_az_152.343_ |
42.048 | 270.0 | node_theta_47.952_az_270_ |
57.941 | 175.158 | node_theta_32.059_az_175.158_ |
[11]:
config.test_configs['dec_2276'].plot_pointings()
[11]:
<PolarAxesSubplot:xlabel='Azimuth'>
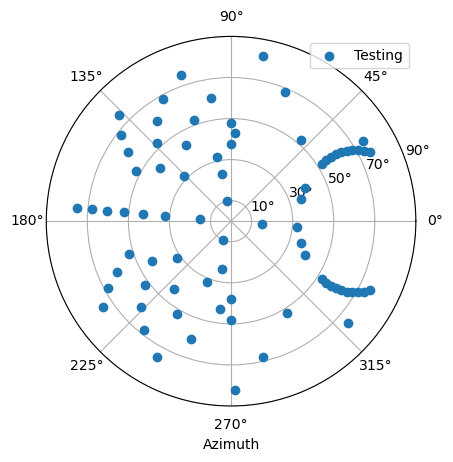
Put them all together#
[12]:
ax = config.test_configs['dec_2276'].plot_pointings(color='black', marker='*')
for dec, cfg in config.train_configs.items():
ax = cfg.plot_pointings(ax=ax, alpha=0.6, s=10)
ax.legend(bbox_to_anchor=(1.1, 1.05))
[12]:
<matplotlib.legend.Legend at 0x7fecc8b0a390>
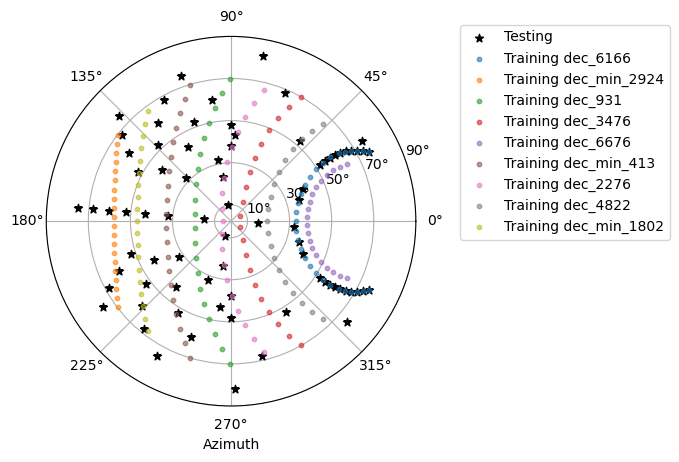
Or in 3D
[13]:
ax = config.test_configs['dec_2276'].plot_pointings(projection='3d', color='black', marker='*')
for dec, cfg in config.train_configs.items():
ax = cfg.plot_pointings(ax=ax, projection='3d', alpha=0.6, s=10)
ax.legend(bbox_to_anchor=(1.1, 1.05))
[13]:
<matplotlib.legend.Legend at 0x7fecc89f8f90>
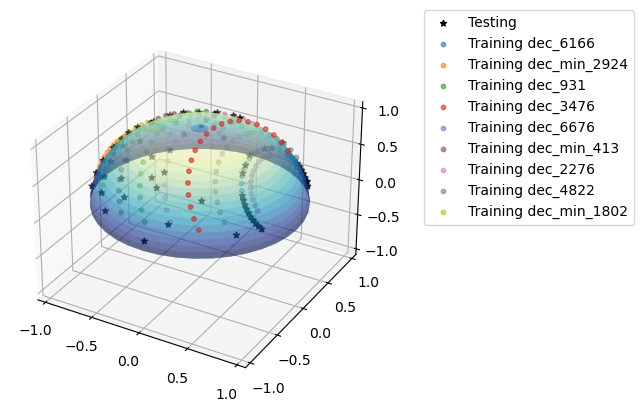
[ ]: